Latest Ignite Release
View on GithubProven Recipes for your React Native apps
Starting from scratch doesn’t always make sense. That’s why we made the Ignite Cookbook for React Native – an easy way for developers to browse and share code snippets (or “recipes”) that actually work.
Spin Up Your App In Record Time
Stop reinventing the wheel on every project. Use the Ignite CLI to get your app started. Then, hop over to the Ignite Cookbook for React Native to browse for things like libraries in “cookie cutter” templates that work for almost any project. It’s the fastest way to get a React Native app off the ground.
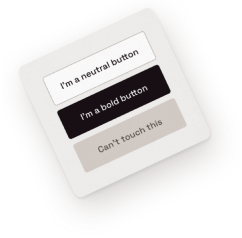
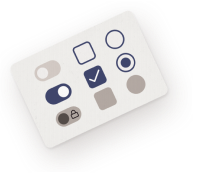
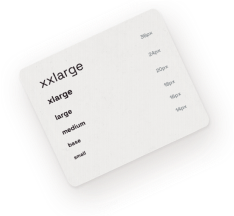
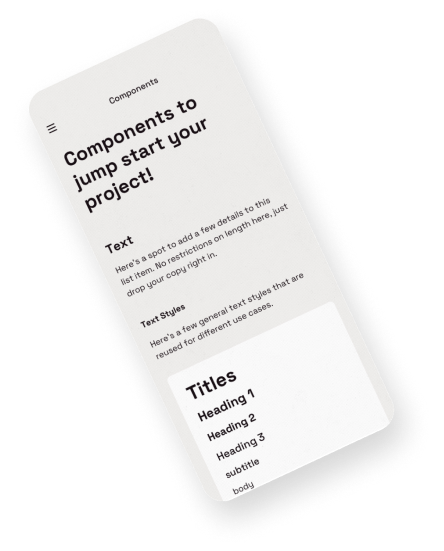
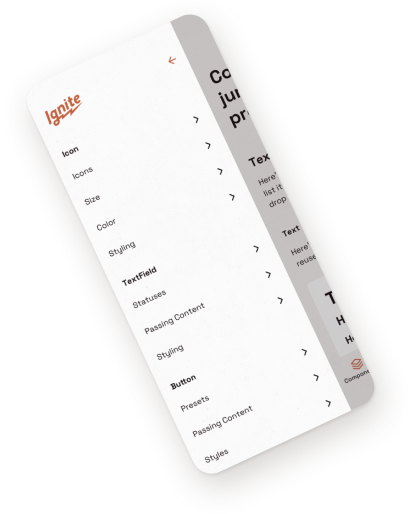
Find Quality Code When You Need It
The popular forum sites are great for finding code until you realize it’s based on an old version of React Native. Ignite Cookbook is a place for recipes that work as of the time they’re published – meaning, it worked when it was posted. And if it ever goes out of date, we’ll make sure the community knows on what version it was last working.
import { ThemeProvider as EmotionThemeProvider } from "@emotion/react"; const EmotionJSThemeProvider = (props: React.PropsWithChildren) => { const { theme } = useAppTheme(); return <EmotionThemeProvider theme={theme}>{props.children}</EmotionThemeProvider>; }; return ( <ThemeProvider value={{ themeScheme, setThemeContextOverride }}> + <EmotionJSThemeProvider> <NavigationContainer ref={navigationRef} theme={navigationTheme} {...props}> <AppStack /> </NavigationContainer> + </EmotionJSThemeProvider> </ThemeProvider> ); import styled from "@emotion/native"; const MyTextComponent = styled.Text` margin: 10px; color: ${({ theme }) => theme.colors.text}; background-color: ${({ theme }) => theme.colors.background}; `; export const MyScreen = (props) => { return ( <MyTextComponent> This text color and background will change when changing themes. </MyTextComponent> ); }; // Override Theme to get accurate typings for your project. import type { Theme as AppTheme } from "app/theme"; import "@emotion/react"; declare module "@emotion/react" { export interface Theme extends AppTheme {} } const { setThemeContextOverride, themeContext } = useAppTheme(); return ( <Button onPress={() => { LayoutAnimation.configureNext(LayoutAnimation.Presets.easeInEaseOut); // Animate the transition setThemeContextOverride(themeContext === "dark" ? "light" : "dark"); }} text={`Switch Theme: ${themeContext}`} /> );
Backed By A Community of React Native Experts
The Ignite Cookbook isn’t just a random group of code snippets. It’s a curated collection of usable code samples that the Infinite Red team’s used in their own React Native projects. Having worked with some of the biggest clients in the tech industry, we know a thing or two about keeping our code to a high standard. You can code confidently!
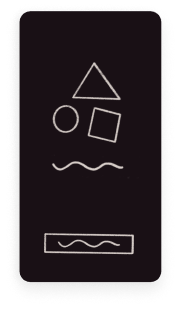
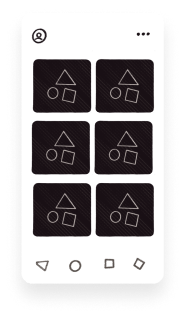
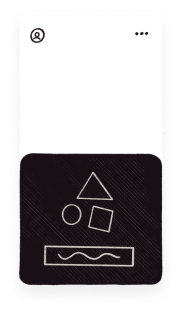
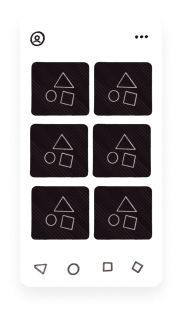
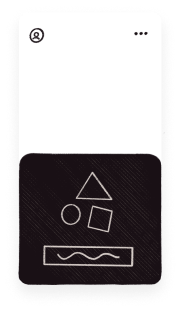
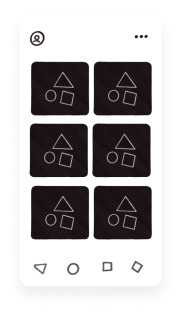
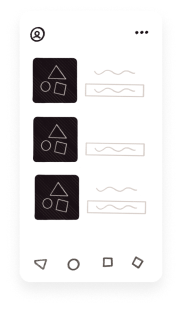
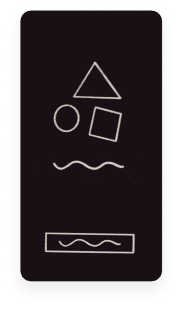
Freshly added to the cookbook
Theming Ignite with Emotion.js
Published on October 2nd, 2024 by Mark Rickert
Theming Ignite with styled-components
Published on October 2nd, 2024 by Mark Rickert
Theming Ignite with Unistyles
Published on October 2nd, 2024 by Mark Rickert
Migrating from i18n-js to react-i18next
Published on September 25th, 2024 by Felipe Peña
View all recipes